Karate DSL is a relatively new tool in the market to perform web services testing. One of the main advantage of this tool is that a tester need not to be a coding expert because it’s built on top of ‘Cucumber’ thus follow ‘Gherkin’ format. See, more details below.
In this guide, we are going to present solutions to perform REST API automation testing using Karate which are easily understandable and quick to implement and use. Well, this is not a complete guide but help you to get the basic details about tool. To learn this tool thoroughly, Go checkout ‘Karate Dsl’ tutorial here.
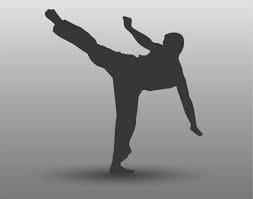
Check out: APACHE HTTPCLIENT TUTORIAL with examples (Latest 2022)
1. Introduction
Karate DSL was released by Intuit as an open source tool to perform API Testing. Those who don’t know coding, this tool will make their life easier.
Key Points:
- It is built on top of Cucumber JVM.
- Tests are easy to write even for non-programmers.
- It supports multi-threaded parallel execution.
- You can generate reports just like any other standard Java project.
- If you are already using Java and Cucumber in your project, then KarateDSL might be a great choice.
2. Using Karate (How To)
In order to start the implementation of Karate DSL based test scripts, we need to set up the environment first. For this reason, this tutorial will explain and help to set up the prerequisite and required dependencies in Eclipse.
Maven based Project:
If you are using Maven as the build tool for you project, use below mentioned dependency and add it into the POM file:
1 2 3 4 5 6 7 8 9 10 11 12 | <dependency> <groupId>com.intuit.karate</groupId> <artifactId>karate-apache</artifactId> <version>0.9.4</version> <scope>test</scope> </dependency> <dependency> <groupId>com.intuit.karate</groupId> <artifactId>karate-junit4</artifactId> <version>0.9.4</version> <scope>test</scope> </dependency> |
Note: If you are going to use Junit-5 then make sure to use karate-junit5 instead of karate-junit4 in the dependency above.
Gradle based Project:
1 2 | testCompile 'com.intuit.karate:karate-junit4:0.9.4' testCompile 'com.intuit.karate:karate-apache:0.9.4' |
3. Project Folder Structure
If you follow the below screenshot, there are 2 main files present in the Karate Project:
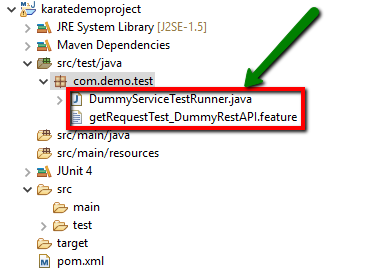
- Feature file (.feature) : Those who worked on cucumber, they must be aware of what this file is all about. Basically, the karate test script has the file extension of .feature which is followed by Cucumber. You are free to organize your files using regular Java package conventions.
- Test Runner file (.java) : Just like Cucumber, you need to use a runner class file to execute the feature files. The only difference you will see unlike cucumber is that you don’t need step definitions in Karate.
If your project is maven based, you generally follow the regular maven based structure in which you keep the source files separately from the *.java files. But, what karate team suggested to keep both files at the same place. If you notice in the above screenshot, both .feature file and .java file are in one package (com.demo.test) inside src/test/java.
Also, apart from these 2 files, there is one more file that you need if your project is maven based and i.e POM.xml. See below screenshot to refer:
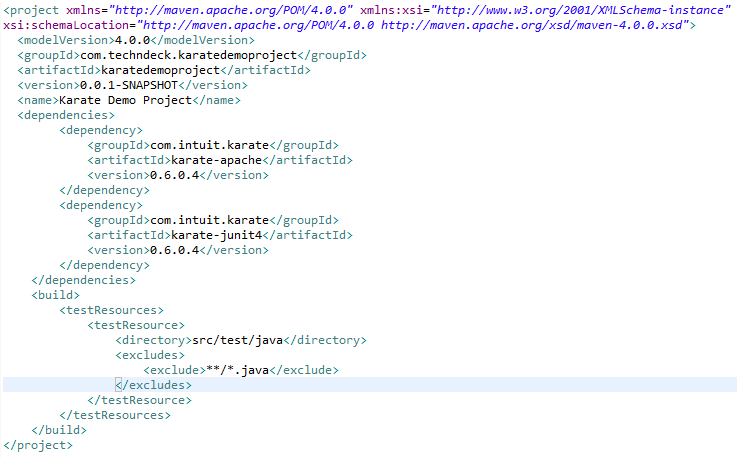
4. Testing HTTP Resources
In this section, we will test the ‘Dummy Sample Rest API’ which is available here. This page contains Fake Online REST API for the testing purpose which are performing various CRUD operations.
4.1 GET Endpoint Request
Let’s take an example of one of the API GET endpoint available at the above-mentioned website which is ‘/employees’. The full-service URL with endpoint is ‘http://dummy.restapiexample.com/api/v1/employees‘.
At the above resource URL, information about all the employees is present and now we are trying to access those employees details in this below example like id, employee_name, employee_age, employee_salary and profile_image.
Here is the code to send the GET request to the above mentioned Service Endpoint:
1 2 3 4 5 6 7 8 9 | Feature: Get Employees Scenario: Get the information of all the employees Given url 'http://dummy.restapiexample.com/api/v1/employees' When method get Then status 200 And print 'Response is: ', response And match response contains {"id": "2091","employee_name": "Wadkar Performance","employee_salary": "700000","employee_age": "29","profile_image": ""} |
Let’s try to understand the code:
1. Specifying the Feature & Scenario (typical cucumber format)
1 2 3 | Feature: Get Employees Scenario: Get the information of all the employees |
2. Setting up Endpoint URI in ‘Given’
1 | Given url 'http://dummy.restapiexample.com/api/v1/employees' |
Endpoint URI is the address to the Resource. And, by this particular line of code, we are specifying to Karate to use “dummy.restapiexample.com/api/v1” as the root URL of the service.
3. Specifying the type of request (GET) in ‘When’ condition
1 | When method get |
4. Checking up Status Code & other Response validations in ‘Then’
1 2 3 | Then status 200 And print 'Response is: ', response And match response contains {"id": "2091","employee_name": "Wadkar Performance","employee_salary": "700000","employee_age": "29","profile_image": ""} |
a. status 200 : It will check the status code coming back from the service is 200
b. print ‘Response is: ‘, response : This line of code will print the response from the service in the console.
c. match response contains {“id”: “2091”,”employee_name”: “Wadkar Performance”,”employee_salary”: “700000”,”employee_age”: “29”,”profile_image”: “”} : This line of code helps to check if the specified content is present in the response or not.
Eclipse Console Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | [{"id":"2091","employee_name":"Wadkar Performance","employee_salary":"700000"...}] 23:40:08.595 [main] DEBUG com.intuit.karate - response time in milliseconds: 379 23:40:08.607 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:40:08.613 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:40:08.615 [main] INFO com.intuit.karate - [print] Response is: [ { "id": "2091", "employee_name": "Wadkar Performance", "employee_salary": "700000", "employee_age": "29", "profile_image": "" }, { "id": "2095", "employee_name": "Amit Negi111", "employee_salary": "123456", "employee_age": "44", "profile_image": "" }, { "id": "2099", "employee_name": "Sowmya", "employee_salary": "0", "employee_age": "25", "profile_image": "" }, ... ] 23:40:08.621 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:40:08.621 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:40:08.622 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 1 Scenarios (1 passed) ... |
4.2 POST Endpoint Request
Let’s take an example of one of the API POST endpoint available at the above-mentioned website which is ‘/create’. The full-service URL with endpoint is ‘http://dummy.restapiexample.com/api/v1/create‘.
At the above resource URL, we are going to submit data in the form of JSON to create an employee.
JSON Request Body:
1 2 3 4 5 | { "name":"Isha", "salary":"5000", "age":"20" } |
Here is the code to send the POST request to the above mentioned Service Endpoint:
1 2 3 4 5 6 7 8 9 10 | Feature: Create Employee Scenario: Verify that a new employee is successfully getting created Given url 'http://dummy.restapiexample.com/api/v1/create' When request { "name":"Isha", "salary":"5000", "age":"20" } And method post Then status 200 And print 'Response is: ', response And match response == {"name": "Isha","salary": "5000","age": "20","id": "2205"} |
Let’s try to understand the code:
1. Specifying the Feature & Scenario (typical cucumber format)
1 2 3 | Feature: Create Employee Scenario: Verify that a new employee is successfully getting created |
2. Setting up Endpoint URI in ‘Given’
1 | Given url 'http://dummy.restapiexample.com/api/v1/create' |
Endpoint URI is the address to the Resource. And, by this particular line of code, we are specifying to Karate to use “dummy.restapiexample.com/api/v1” as the root URL of the service.
3. Specifying the ‘json’ formatted employee data that need to be sent to the service to create the employee
1 | When request { "name":"Isha", "salary":"5000", "age":"20" } |
4. Specifying the type of request (POST)
1 | And method post |
5. Checking up Status Code & other Response validations in ‘Then’
1 2 3 | Then status 200 And print 'Response is: ', response And match response == {"name": "Isha","salary": "5000","age": "20","id": "2205"} |
a. status 200 : It will check the status code coming back from the service is 200
b. print ‘Response is: ‘, response : This line of code will print the response from the service in the console.
c. match response == {“name”: “Isha”,”salary”: “5000”,”age”: “20”,”id”: “2205”} : This line of code helps to check if the response coming from the service exactly matches with the specified expected content.
Eclipse Console Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | ... {"name":"Isha","salary":"5000","age":"20","id":"2205"} 23:40:09.068 [main] DEBUG com.intuit.karate - response time in milliseconds: 371 23:40:09.076 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:40:09.082 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:40:09.082 [main] INFO com.intuit.karate - [print] Response is: { "name": "Isha", "salary": "5000", "age": "20", "id": "2205" } 1 Scenarios (1 passed) ... |
4.3 PUT Endpoint Request
Let’s take an example of one of the API PUT endpoint available at the above-mentioned website which is ‘/update/{id}’. The full-service URL with endpoint is ‘http://dummy.restapiexample.com/api/v1/update/{id}’.
At the above resource URL, we are going to submit data in the form of JSON to update an existing employee which is having ‘id’ as ‘4710’.
NOTE: This ‘id’ belongs to the employee which is generated during the POST call to create the employee. So, this id : 4710 generated for me when I had executed the create employee using the POST call. In order to get yours, first execute the POST Request call available at this link and grab the ‘id‘ from the response and use it in your PUT Request Test below in place where I am using ‘4710’.
JSON Request Body:
1 2 3 4 5 | { "name":"put_test_employee", "salary":"1123", "age":"23" } |
Here is the code to send the PUT request to the above mentioned Service Endpoint:
1 2 3 4 5 6 7 8 9 10 | Feature: Update Employee Scenario: Verify that the employee information is successfully updated Given url 'http://dummy.restapiexample.com/api/v1/update/4710' When request { "name":"put_test_employee", "salary":"1123", "age":"23" } And method put Then status 200 And print 'Response is: ', response And match response == {"name": "put_test_employee","salary": "1123","age": "23"} |
Let’s try to understand the code:
1. Specifying the Feature & Scenario (typical cucumber format)
1 | Feature: Update Employee Scenario: Verify that the employee information is successfully updated |
2. Setting up Endpoint URI in ‘Given’
1 | Given url 'http://dummy.restapiexample.com/api/v1/update/4710' |
Endpoint URI is the address to the Resource. And, by this particular line of code, we are specifying to Karate to use “dummy.restapiexample.com/api/v1” as the root URL of the service.
3. Specifying the ‘json’ formatted employee data that need to be sent to the service to update the employee
1 | When request { "name":"put_test_employee", "salary":"1123", "age":"23" } |
4. Specifying the type of request (POST)
1 | And method put |
5. Checking up Status Code & other Response validations in ‘Then’
1 2 3 | Then status 200 And print 'Response is: ', response And match response == {"name": "put_test_employee","salary": "1123","age": "23"} |
a. status 200 : It will check the status code coming back from the service is 200
b. print ‘Response is: ‘, response : This line of code will print the response from the service in the console.
c. match response == {“name”: “put_test_employee”,”salary”: “1123”,”age”: “23”} : This line of code helps to check if the response coming from the service exactly matches with the specified expected content.
Eclipse Console Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | ... {"name":"put_test_employee","salary":"1123","age":"23"} 23:31:29.124 [main] DEBUG com.intuit.karate - response time in milliseconds: 384 23:31:29.135 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:31:29.140 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:31:29.140 [main] INFO com.intuit.karate - [print] Response is: { "name": "put_test_employee", "salary": "1123", "age": "23" } 23:31:29.141 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:31:29.141 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:31:29.141 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 1 Scenarios (1 passed) ... |
4.4 DELETE Endpoint Request
Let’s take an example of one of the API DELETE endpoint available at the above-mentioned website which is ‘/delete/{id}’. The full-service URL with endpoint is ‘http://dummy.restapiexample.com/api/v1/delete/{id}’.
At the above resource URL, we are going to make a delete call to remove an existing employee who is having ‘id’ as ‘11400’.
NOTE: This ‘id’ belongs to the employee which is generated during the POST call to create the employee. So, this id : 11400 generated for me when I had executed the create employee using the POST call. In order to get yours, first execute the POST Request call available at this link and grab the ‘id’ from the response and use it in your DELETE Request Test in place where I am using ‘11400’.
Here is the code to send the DELETE request to the above mentioned Service Endpoint:
1 2 3 4 5 6 7 8 | Feature: Delete Employee Scenario: Verify that the existing employee is successfully getting deleted Given url 'http://dummy.restapiexample.com/api/v1/delete/11400' And method delete Then status 200 And print 'Response is: ', response |
Let’s try to understand the code:
1. Specifying the Feature & Scenario (typical cucumber format)
1 2 3 | Feature: Delete Employee Scenario: Verify that the existing employee is successfully getting deleted |
2. Setting up Endpoint URI in ‘Given’
1 | Given url 'http://dummy.restapiexample.com/api/v1/delete/11400' |
Endpoint URI is the address to the Resource. And, by this particular line of code, we are specifying to Karate to use “dummy.restapiexample.com/api/v1” as the root URL of the service.
3. Specifying the type of request (DELETE) in ‘When’ condition
1 | When method delete |
4. Checking up Status Code & other Response validations in ‘Then’
1 2 | Then status 200 And print 'Response is: ', response |
a. status 200 : It will check the status code coming back from the service is 200
b. print ‘Response is: ‘, response : This line of code will print the response from the service in the console.
Eclipse Console Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | ... {"success":{"text":"successfully! deleted Records"}} 23:31:27.540 [main] DEBUG com.intuit.karate - response time in milliseconds: 1788 23:31:27.657 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:31:27.669 [main] DEBUG com.jayway.jsonpath.internal.path.CompiledPath - Evaluating path: $ 23:31:27.669 [main] INFO com.intuit.karate - [print] Response is: { "success": { "text": "successfully! deleted Records" } } 1 Scenarios (1 passed) ... |
5. Resources
intuit/karate: Test Automation Made Simple – GitHub
Testing Web Services with KARATE...!!! Share on XCheckout other useful tutorials (competitors of Karate), take a look: